高级IO函数
高级IO函数
基本的GCI服务器
CGI(Common Gateway Interface)服务器是一种通过 Web 服务器执行外部程序的标准接口。它允许 Web 服务器调用外部程序来处理客户端的请求,并将程序的输出发送回客户端浏览器。CGI 是连接 Web 服务器和其他软件(通常是动态生成的程序或脚本)的桥梁。
基本上,当 Web 服务器接收到一个对动态内容的请求时,它可以调用与之相关联的 CGI 脚本或程序,将用户的请求传递给该程序进行处理。该程序生成动态内容,然后将其输出返回给 Web 服务器,最终发送到用户的浏览器。
一些关键点和特性:
- 动态内容生成: CGI 允许服务器调用外部程序来生成动态内容,而不是直接返回静态文件。
- 编程语言无关性: CGI 接口是独立于编程语言的,因此可以使用多种编程语言编写 CGI 脚本,包括但不限于 Perl、Python、C、C++等。
- 处理表单数据: CGI 可以用于处理来自 HTML 表单的数据。用户提交表单后,服务器可以调用 CGI 脚本来处理表单数据并生成相应的响应。
- 与 Web 服务器的通信: CGI 脚本与 Web 服务器通过环境变量和标准输入输出进行通信。
- 安全性考虑: 由于 CGI 允许执行外部程序,必须小心处理用户输入,以防止潜在的安全漏洞。
虽然 CGI 曾经是 Web 开发的主要方式,但随着时间的推移,其他技术和方法,如 FastCGI、mod_perl、PHP、ASP.NET 等,已经逐渐取代了纯粹的 CGI 模型,提供了更高效和更强大的动态内容生成机制。
将服务器标准输出重定向到客户端:
1 |
|
关闭标准输出(STDOUT_FILENO):
1 | close(STDOUT_FILENO); |
close
函数用于关闭文件描述符。在这里,关闭标准输出文件描述符STDOUT_FILENO
,这是与标准输出相关联的文件描述符。
复制连接套接字到标准输出:
1 | dup(connfd); |
dup
函数用于复制文件描述符。在这里,将连接套接字的文件描述符connfd
复制到标准输出的文件描述符STDOUT_FILENO
。这样,标准输出将与连接套接字相关联。- dup函数会创建一个新的文件描述符,该描述符和原文件描述符指向相同的文件‘管道或网络连接
- 现在,
printf
函数将数据写入标准输出,实际上是写入了连接套接字,因为标准输出已经被重定向到了连接套接字。
测试:
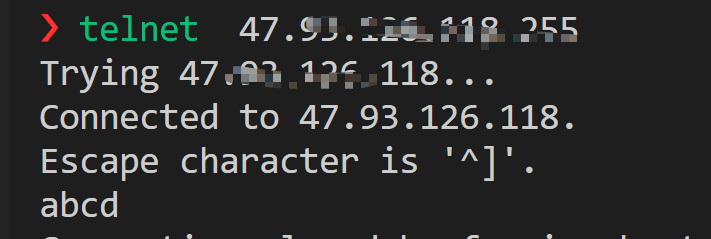
web服务器上集中写
readv函数是将数据从文件描述符读到分散的内存块中,writev函数将分散内存数据一并写入文件描述符中
1 |
|
send( connfd, header_buf, strlen( header_buf ), 0 );
:
send
函数用于通过指定的套接字 (connfd
) 发送数据。header_buf
是包含待发送数据的缓冲区的地址。strlen(header_buf)
是待发送数据的长度,使用strlen
函数计算字符串的长度。0
是标志参数,表示不使用特殊的发送选项。
测试:

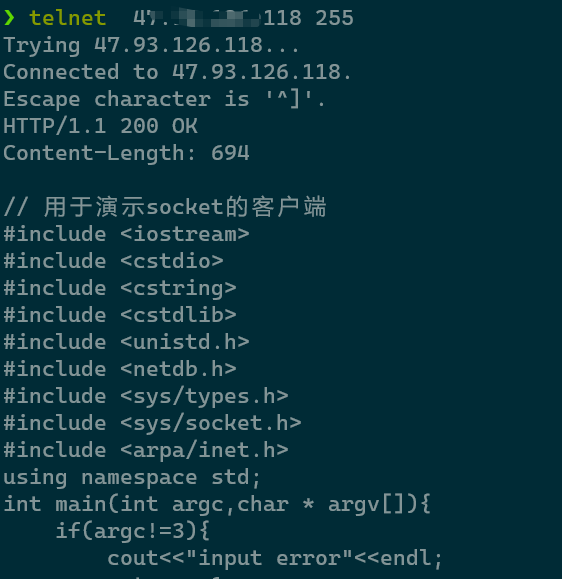
sendfile函数传输文件
修改schedule函数
1 |
|
与之前的方法比,不用分配缓存就完成了文件的传输 。实验效果和上面一样
用splice函数实现回射服务器
将客户端发送的数据原样返回客户端
1 |
|
这段代码涉及到 Linux 中的管道(pipe
)和
splice
系统调用,用于实现零拷贝数据传输。
int pipefd[2];
定义了一个整型数组
pipefd
用于存储管道的两个文件描述符。使用
pipe
函数创建了一个无名管道。pipefd[0]
用于读取,pipefd[1]
用于写入使用
splice
将connfd
(客户端连接套接字)的数据传输到管道的写入端 (pipefd[1]
)。32768
是指定的最大传输字节数。SPLICE_F_MORE | SPLICE_F_MOVE
是传输标志,其中SPLICE_F_MORE
表示可能还有更多的数据,SPLICE_F_MOVE
:合适的话按整页内存移动数据
实验效果:
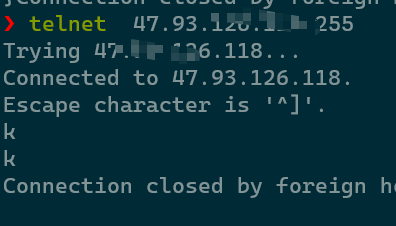
TEE 同时输出数据到终端和文件
tee在两个管道文件描述符之间复制数据,零拷贝
1 |
|